Forum scraping: A quick guide for developers
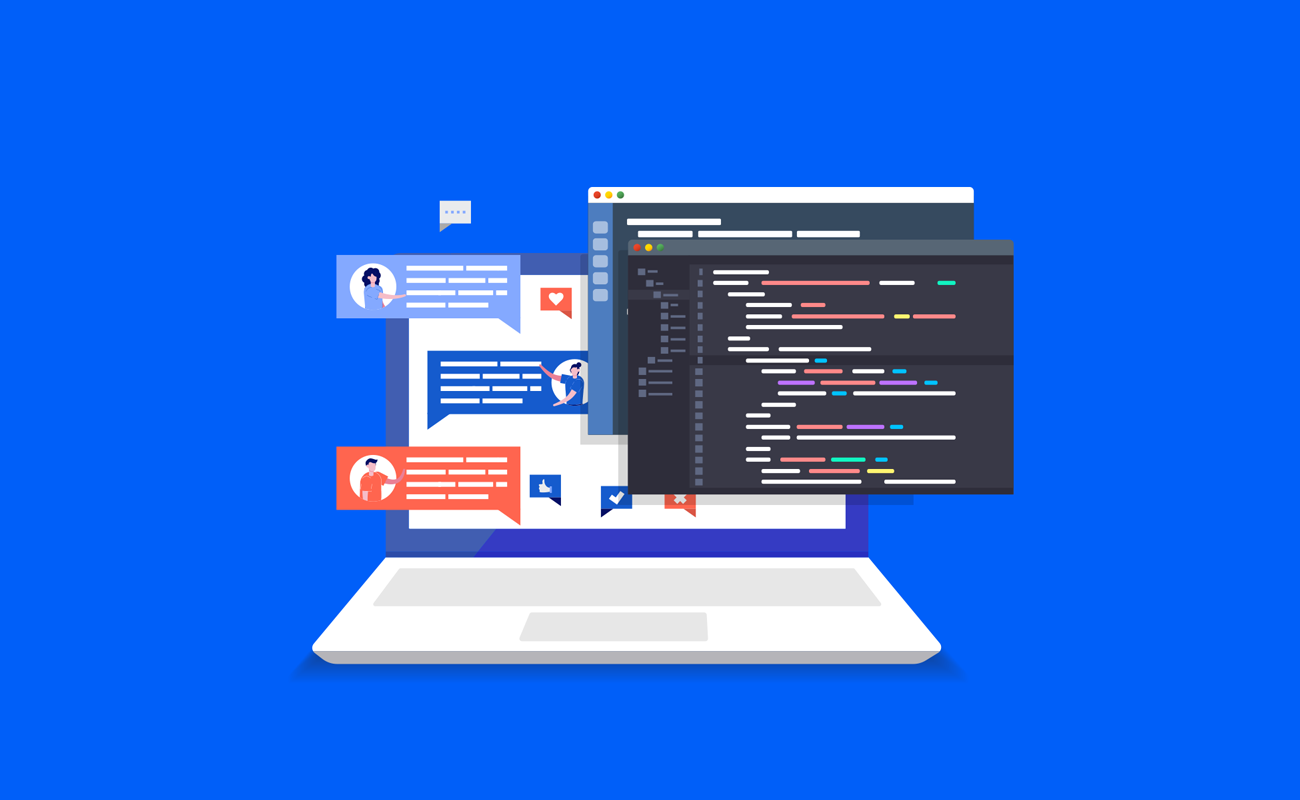
This is your guide if you're a senior developer aiming to harness the untapped data reservoirs lurking in online forums.
Forum scraping is no longer a fringe activity; it's necessary for data-driven decision-making in various sectors, from business analytics to academic research. This guide is a deep dive into forum scraping, covering everything from the Python libraries you'll need to the ethical considerations you can't afford to ignore.
What Should Developers Expect from Web Scraping Forums?
For developers, forum scraping is a goldmine of opportunities beyond data collection. It's about turning unstructured, user-generated content into structured data that can be leveraged for many applications. Here's how you can use forum scraping to your advantage:
Business Analytics
If you're working on a business analytics project, scraping forums can provide invaluable data on consumer sentiment. This data can be processed and analyzed to inform business strategies. For instance, you can feed this data into machine learning models to predict future consumer behavior, product success, or market trends.
Real Estate and Market Research
Developers working in sectors like real estate can scrape forums to gather insights on real estate listings, property values, neighborhood safety, and other factors influencing buying decisions.
This data can be integrated into larger machine-learning models or analytics tools that help companies or consumers make informed decisions.
NLP and Machine Learning Projects
Forum scraping is particularly beneficial if you work on Natural Language Processing or other machine learning projects. The extracted data can be used for sentiment analysis, chatbot training, or even to build recommendation systems.
Pre-requisites and Tools for Forum Scraping
Before diving into forum scraping, it's essential to have a few things in place:
Programming Language: Python is highly recommended due to its extensive libraries and community support for web scraping. Ensure you grasp Python basics well, including loops, functions, and libraries.
HTML and CSS Knowledge: Understanding the structure of web pages is crucial for effective scraping. Familiarize yourself with HTML tags, attributes, and CSS selectors to navigate and parse web pages.
Text Editor: A robust text editor like Visual Studio Code or Sublime Text is essential for coding. These editors offer syntax highlighting and auto-completion features, which can speed up the development process.
Libraries: Libraries like Requests, BeautifulSoup,
asyncio
andaiohttp
are fundamental for web scraping. These libraries help send HTTP requests, process HTML content, and handle asynchronous tasks.
Setting Up Your Development Environment
The initial phase of your forum scraping expedition involves configuring your development environment. To guide you through this:
Python Installation: Navigate to Python's official portal and download the latest stable release. Execute the installer and abide by the on-screen directives.
Text Editor Configuration: Opt for a text editor such as Visual Studio Code, renowned for its integrated Python support.
Library Installation: Fire up your terminal or command prompt and execute these commands to incorporate the essential libraries:
1Bashpip install requests beautifulsoup4 asyncio aiohttp
Verify Installation: To ensure everything is set up correctly, open your text editor and try importing the libraries:
1Pythonimport requests from bs4 import BeautifulSoup import asyncio import aiohttp
If there are no errors, you're good to go!
The Anatomy of a Forum Page
Understanding the anatomy of a forum page is crucial for effective scraping. A typical forum contains multiple data layers, from categories to individual threads and posts. Each of these layers has its HTML structure, often nested within each other.
For instance, a thread list might be contained within a <div> tag, with each thread represented by an <a> tag.
Posts within a thread could be listed under another <div> tag, each with its unique identifier. Metadata like timestamps, user names, and post counts are usually found in specific HTML classes or IDs. Navigating this complex structure is critical to extracting the data you need.
Asynchronous Web Scraping
Asynchronous web scraping is essential when dealing with forums that have multiple pages or threads. Traditional synchronous scraping would fetch one page at a time, making the process time-consuming, while asynchronous scraping accelerates the process. But it comes with challenges: Handling rate limits is a significant concern, as too many requests too quickly can ban your IP address from the forum or website. There's also the potential to face CAPTCHAs or other anti-bot measures, so developers must be cautious and incorporate strategies to mimic human behavior.
On the other hand, asynchronous scraping allows you to fetch multiple pages simultaneously, significantly speeding up the data extraction process.Here's how to set up asynchronous web scraping using Python's asyncio
and aiohttp
libraries:
1.Import Libraries: First, import the necessary libraries.
1Pythonimport asyncio import aiohttp
2.Create an Asynchronous Function: Create a function to fetch a webpage asynchronously.
1Pythonasync def fetch_page(session, url):2 async with session.get(url) as response:3 return await response.text()
3.Create the Main Function: Create the primary function to manage the scraping.
1Pythonasync def main(): async with aiohttp.ClientSession() as session: html = await fetch_page(session, 'https://example-forum.com') # Parsing logic here
Run the Asynchronous Loop: Finally, run the asynchronous event loop.
1Pythonloop = asyncio.get_event_loop()2loop.run_until_complete(main())
By following these steps, you can set up an asynchronous web scraping environment that can efficiently handle the large amount of data typically found in forums.
Data Storage and Structured Formats
After successfully scraping the forum data, the next crucial step is to store it in a structured format for easy retrieval and analysis.
Modern data storage solutions besides traditional formats like JSON and SQL can be beneficial. NoSQL databases, such as MongoDB, can be particularly adept at handling unstructured or semi-structured data that might emerge from forum scrapes. Moreover, cloud storage solutions like Amazon S3 or Google Cloud Storage can be helpful to for extensive datasets that require scalable and accessible storage options.
JSON
The JSON (JavaScript Object Notation) format is lightweight and easy to read. Python's JSON library can be used to serialize the scraped data into a JSON file. Here's a quick example:
1Jsonimport json23scraped_data = {'thread_title': 'Example', 'posts': ['post1', 'post2']}45with open('scraped_data.json', 'w') as f:6 json.dump(scraped_data, f)
SQL Database
A relational database like MySQL or SQLite can be more appropriate for complex data structures. Python's SQLAlchemy library can be used to interact with SQL databases. Here's a simple code snippet to insert data into an SQLite database:
1Sqlfrom sqlalchemy import create_engine23engine = create_engine('sqlite:///scraped_data.db')45engine.execute("INSERT INTO forum_data (thread_title, post) VALUES ('Example', 'post1')")
NoSQL Databases
NoSQL databases, such as MongoDB, can be invaluable for unstructured or semi-structured data. Here's a basic way to insert data into MongoDB using the pymongo
library.
1Pythonfrom pymongo import MongoClient23client = MongoClient('localhost', 27017)4db = client['forum_data_db']5collection = db['forum_data']67scraped_data = {'thread_title': 'Example', 'posts': ['post1', 'post2']}8collection.insert_one(scraped_data)
Cloud Storage Solutions:
Cloud storage solutions like Amazon S3 can be used to store large datasets. Using the boto3
library, you can upload scraped data to an S3 bucket.
1Pythonimport boto323s3 = boto3.resource('s3')4s3.meta.client.upload_file('scraped_data.json', 'mybucket', 'forum_data/scraped_data.json')
Choosing the correct storage format depends on your specific needs, such as the complexity of the data and the type of analysis you plan to perform.
Natural Language Processing for Forum Data
Forums are a treasure trove of user-generated content, filled with emotions, abbreviations, slang, and diverse linguistic structures. This complexity is why Natural Language Processing (NLP) techniques are essential for forum data. Processing and analyzing this diverse range of text accurately can offer profound insights into user sentiments, preferences, and behavior patterns.
Whether it's sentiment analysis, topic modeling, or text summarization, NLP can be beneficial.Python offers several libraries for NLP, including:
NLTK: The Natural Language Toolkit (NLTK) is a comprehensive library for NLP. It offers various text-processing algorithms, from tokenization to complex machine-learning models.
spaCy: spaCy is another powerful library for advanced NLP tasks known for its speed and accuracy.
Here's a simple example using spaCy for sentiment analysis:
1Pythonimport spacy2from textblob import TextBlob34nlp = spacy.load('en_core_web_sm')56doc = nlp("The product is great")78blob = TextBlob(doc.text)910print(blob.sentiment.polarity)
Once the data is stored, you can leverage NLP techniques to extract insights.
Understanding Performance Metrics
Understanding performance metrics is crucial for developers engaged in forum scraping. Different libraries and techniques can impact speed, efficiency, and resource utilization.
Library Comparison:
Libraries like requests, BeautifulSoup, Scrapy, and aiohttp
are commonly used for web scraping. Each has pros and cons regarding speed, ease of use, and flexibility.
Technique Comparison:
Techniques like synchronous vs. asynchronous scraping, single vs. multi-threading, and API endpoints vs. scraping HTML directly can affect performance.
Benchmarking:
Conducting benchmarks for different libraries and techniques can provide empirical data on their performance. Metrics to consider include request latency, data processing time, and memory usage.
Most Popular Performance Metrics in Forum Scraping
Request Latency: The time it takes to send a request and receive a response is a critical metric. Lower latency is generally better, especially for large-scale scraping projects.
Data Processing Time: The time required to process and structure the scraped data can vary depending on the library and technique. Faster data processing times are generally more desirable.
Memory Usage: Efficient memory usage is crucial, especially when scraping large forums. Some libraries are more memory-efficient than others, which can be a deciding factor for large-scale projects.
CPU Utilization: High CPU usage can bottleneck web scraping tasks. Monitoring and optimizing CPU utilization can lead to more efficient scraping operations.
Conclusion
Navigating the world of forum scraping is indispensable for the modern senior developer in a data-centric landscape. This guide has endeavored to illuminate the nuances and intricacies of extracting valuable data from online forums.
It's not merely about scraping; it's about transforming raw, unstructured content into actionable insights. From data storage options like JSON and SQL to leveraging advanced NLP techniques for profound understanding, the success of forum scraping hinges on mastering its multifaceted elements.
FAQs
How do web scrapers work?
Web scrapers send HTTP requests to a target website and then process the HTML content to extract structured data. This is particularly useful for gathering data from websites like forums, where user-generated content is abundant.
What is the role of artificial intelligence in web scraping?
Artificial intelligence can significantly enhance the capabilities of a web scraper. For example, machine learning algorithms can identify patterns or trends in the scraped data, providing more valuable insights.
How do I scrape a site for structured data?
To scrape a site for structured data, you must use a programming language like Python and libraries such as BeautifulSoup or Scrapy. These tools allow you to send HTTP requests and parse HTML content to extract the needed data.
What is web data extraction?
Web data extraction involves pulling specific data from websites. This is often done through web scraping techniques, where HTTP requests are sent to a website, and the returned HTML content is parsed to extract structured data.
What are the basics of web scraping?
The basics of web scraping involve understanding HTML structure, sending HTTP requests to fetch web pages, and then parsing the HTML to extract the data you need. Libraries like Requests and BeautifulSoup are commonly used for these tasks.
What is the difference between web crawling and data scraping?
Web crawling is navigating a website to identify the pages you want to scrape. On the other hand, data scraping involves extracting data from these web pages.